Set up React, TypeScript and Tailwind in Vite
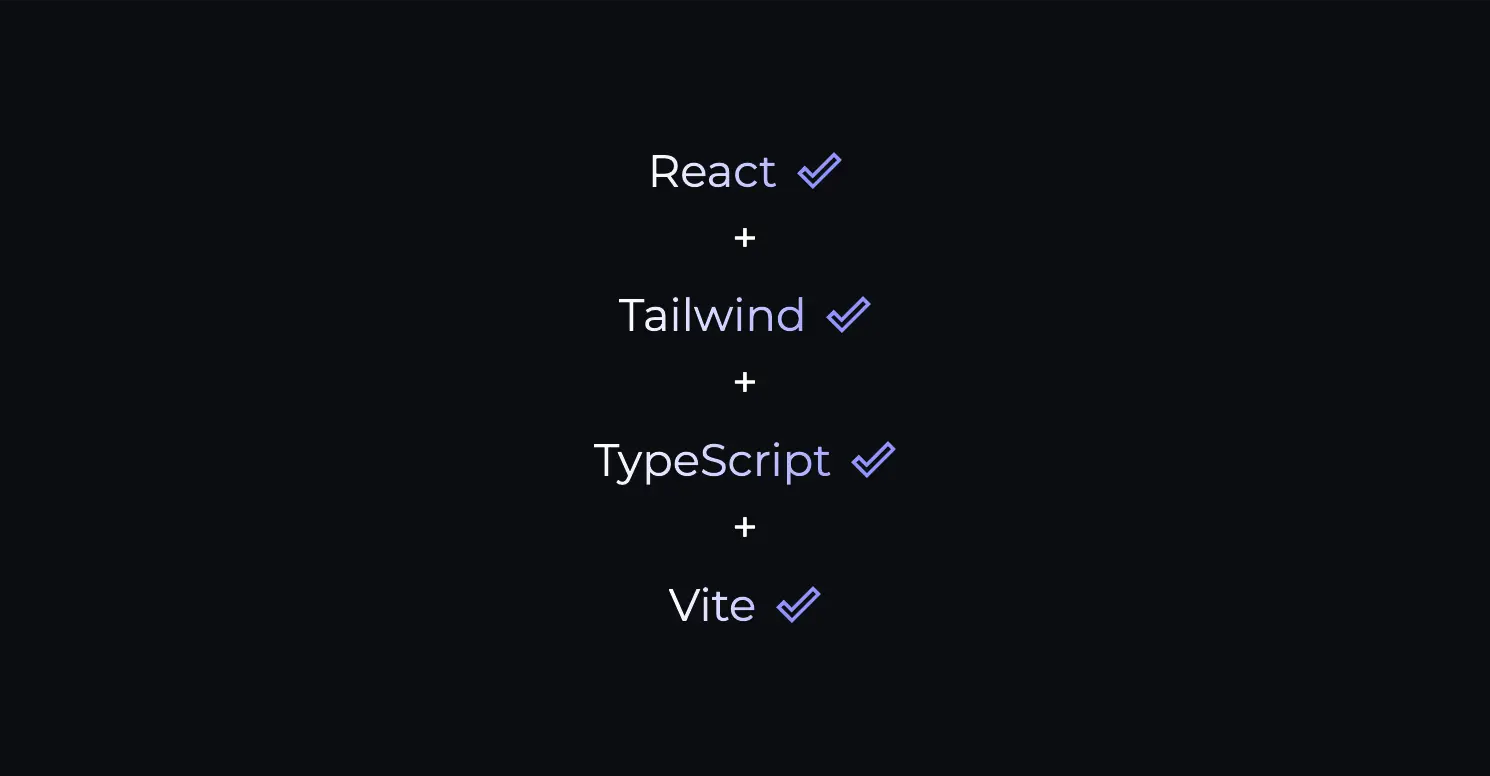
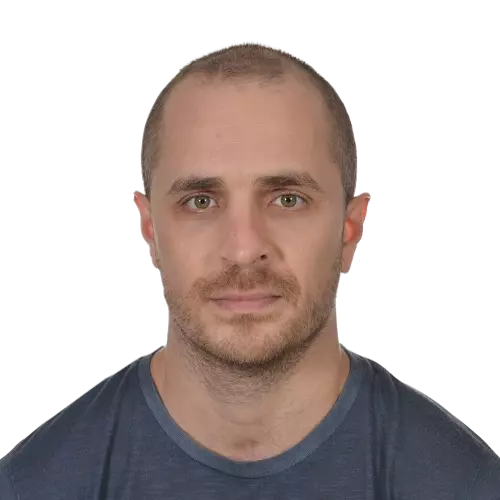
In this article, we will set up a development environment for a react project using Vite, React, TypeScript and Tailwind.
This is probably the most used boilerplate code when it comes to react apps nowadays.
Create the project
Open your terminal of choice and go to the repo where you want to create your project.
Once there, use the following command to create the project:
npm create vite@latest
You will be prompted the following:
- Project name:
my-vite-project
- Select a framework:
React
- Select a variant:
TypeScript
Now go to this newly created repo, install dependencies and start the development server:
cd my-vite-project && npm i && npm run dev
Your project should be up and running at localhost:5173 ↗
Install Tailwind CSS
Time to install tailwind and some other required dependencies
npm i -D tailwindcss postcss autoprefixer
This command means following:
-D
- install the following as dev-dependenciestailwind
- Tailwind CSS frameworkpostcss
- Tool for transforming Tailwind directives into fully generated, optimized stylesautoprefixer
- PostCSS plugin that automatically adds vendor prefixes to your CSS, ensuring compatibility across different browsers
Generate Tailwind configuration files
To start using the tailwind, we need to create configuration files, namely tailwind.config.js
and postcss.config.js
.
We can create them manually, but why would we, when there’s a command which will do that for us:
npx tailwind init -p
Configure Tailwind source paths
In this step we specify the paths of files which will contain tailwind classes, otherwise the styles won’t be applied.
We do that by adding "./index.html"
and "./src/**/*.{js,ts,jsx,tsx}"
to content
array in tailwind.config.js
/* tailwind.config.js */
/** @type {import('tailwindcss').Config} */
export default {
content: ["./index.html", "./src/**/*.{js,ts,jsx,tsx}"] // <---
theme: {
extend: {},
},
plugins: [],
}
Add @tailwind
directives
Add these directives to the top of your index.css
file (or what ever your root CSS file is)
@tailwind base;
@tailwind components;
@tailwind utilities;
This directive imports Tailwind’s pre-designed layers (base, components, and utilities) into your CSS file.
@tailwind base;
- Imports base styles (resets and foundational styles)@tailwind components;
- Imports reusable components (pre-designed classes)@tailwind utilities;
- Imports utility classes (spacing, text sizes, colors, etc.)
Start using Tailwind
Open your App.tsx
file and start using tailwind classes
function App() {
return <h1 className="text-red-500 bg-gray-800">My Vite Project</h1>;
}
export default App;
Happy coding! 🫡
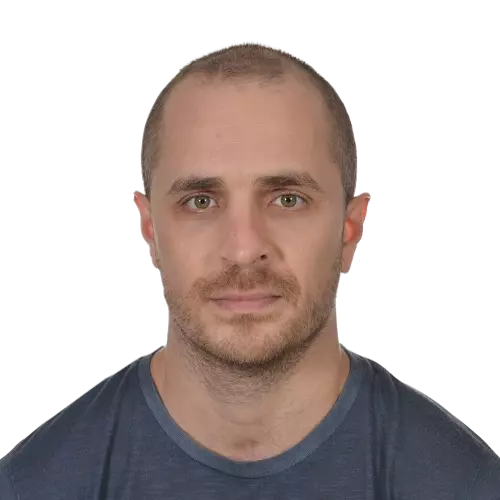